100 Days of Code - Week 05: Days 120-125 (6 Days this week!)
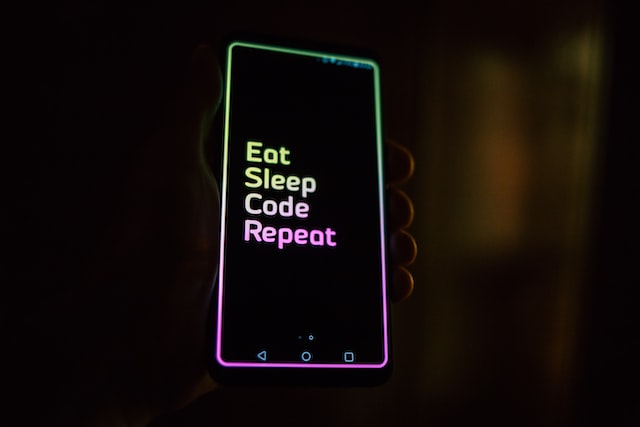
Mar 13, 2023
Finish UI Design, Work on Command Line Skills, and Diving Back Into Javascript!
This is a daily record of my second iteration of 100 Days of Code. This time I'm (usually) doing 5 days a week instead of 7. This is week 05 of 20 weeks. I finished the Scrimba UI Design course, started Wes Bos' Command Line and Flexbox courses, and spent sometime in my Exploring CSS Layouts repo. I also dived back into Javascript on Thursday reviewing constructor functions, methods and Object.assign(). On Friday I updated the design and deployed my Yarn Help! project to my own domain at https://yarnhelp.app. Finally on Saturday I worked extensively on the Docs, including plans for future expansion.
Day 120 | 2023-03-13 | Monday | Focus: UI Design |
Main Resource: Scrimba UI Design Course https://scrimba.com/learn/designbootcamp
Completed:
- Finished UI Animations
- Started final UI Design Course project - to "Defining the Hero Section CSS part 2"
Refection: The animations module wasn't anything new. It was just a colour transform on a single button. I'll need to delve into this deeper at some point. I love design and css, but I'm getting bored; it's time to delve into Javascript again! I really want to apply all this and upskill my Javascript for yarnhelp.app
Day 121 | 2023-03-13 | Tuesday | Focus: UI Design & Command Line |
Main Resources:
Completed:
- ** Finished UI Design Course!**
- Started Wes Bos' Command Line Power User course
UI Design Course Certificate
Refections and Notes for UI Design: Can do css descendent selectors 3 deep not just two deep like this:
/* to target a image(s) inside of an li inside of a list */
ul.features li img {
width: 40px;
}
To make an svg into a watermark:
.fern {
position: absolute;
bottom: 0;
left: 0;
z-index: 1;
width: 80%;
opacity: .1;
}
To have three items in a media query (eg small tablet) align with two on top and one centred below:
.featured-cabins,
.features,
.testimonials /* testimonials fits well three items across so there's no need to apply the li:last-child rules below */
{
display: grid;
grid-template-columns: repeat(auto-fit, minmax(min(120px, 100%), 1fr));
grid-gap: 1rem;
justify-content: space-evenly;
}
/* Just using .features:last-child doesn't work because we're looking for the last li not the last .features */
.featured-cabins li:last-child,
.features li:last-child
{
grid-column: 1 / span 2;
max-width: 50%;
justify-self: center;
}
/* Note: .testimonials fits well three items across so there's no need to apply the li:last-child rules above */
- At some point I should review/get more into GSAP GreenSock Animation Platform: https://greensock.com/get-started/
Reflections and Notes for Command Line Course:
- OhMy zsh is a lifesaver!
- This along with sudo was how I could open the .zshrc file and get around the "permission denied" issue in MacOS.
- Apparently I have Python 3.8.3 installed. (Current release is 3.11.1)
- #UI-design-ideas the https://ohmyz.sh/ site has a nice colour animation on the themes icon. :-)
Day 122 | 2023-03-15 | Wednesday | Focus: Flexbox |
Main Resources:
- WesBos What the Flexbox Course
- My Exploring CSS Layouts repo
Completed:
- What the Flexbox to video 11 of 20: *"Finally understanding Flexbox flex-grow, flex-shrink and flex-basis" **
- Added a flexbox page and .css to repo
Refection: Tired today, but this I can do. Need to fix the github issues with my repo. Could use the grid page as a starting point for yarnhelp.app
Day 123 | 2023-03-16 | Thursday | Focus: Javascript - Review Constructor Functions & Methods |
Main Resources:
- Scrimba Frontend Developer Career Path Module: Next Level Javascript
- Referring back to the existing yarnhelp github repo to see how/if I can apply this to any parts of the short or medium term plans for the site - and actually getting it live on yarnhelp.app
Completed:
- Frontend Developer Career Path - Next Level Javascript up to Object.assign()
Refections and Notes:
- Variable names: This is why I've always used "01" instead of just "1" for numbers. I spent 20minutes trying to see what I was missing/had forgotten about constructor functions with the code below. ... Only to finally figure out that "hotel1" was misspelled "hote1".
// Code and challenge provided on Scrimba
const hotel1 = {
name: 'Safari View',
rooms: 30,
stars: '⭐⭐⭐⭐⭐',
costPerNightAdult: 240,
}
const hotel2 = {
name: 'Leopard Mansion',
rooms: 96,
stars: '⭐⭐⭐',
costPerNightAdult: 120,
}
/*
Challenge:
1. Create a constructor function called NationalParkHotels.
2. Have it take in "data" as a parameter
3. Assign the data to "this"
4. Log out the result of creating an instance of
NationalParkHotels for each hotel.
*/
// Code and console output with spelling error:
function NationalParkHotels(data){
this.name = data.name
this.rooms = data.rooms
this.stars = data.stars
this.costPerNightAdult = data.costPerNightAdult
}
const safariView = new NationalParkHotels(hote1)
const leopardMansion = new NationalParkHotels(hotel2)
console.log(safariView)
// console output
ReferenceError: hote1 is not defined (/index.js:22)
// Code and console output after fixing spelling error:
function NationalParkHotels(data){
this.name = data.name
this.rooms = data.rooms
this.stars = data.stars
this.costPerNightAdult = data.costPerNightAdult
}
const safariView = new NationalParkHotels(hotel1)
const leopardMansion = new NationalParkHotels(hotel2)
console.log(safariView)
console.log(leopardMansion)
// console output after fixing spelling error:
NationalParkHotels {name:"Safari View", rooms:30, stars:"⭐⭐⭐⭐⭐", costPerNightAdult:240}
NationalParkHotels {name:"Leopard Mansion", rooms:96, stars:"⭐⭐⭐", costPerNightAdult:120}
Methods on a Constructor Function Example
function NationalParkHotels(data){
this.name = data.name
this.rooms = data.rooms
this.stars = data.stars
this.costPerNightAdult = data.costPerNightAdult
this.costPerNightChild = data.costPerNightChild
this.summariseHotel = function(){
console.log(`A one night stay
at the ${this.name}for two adults and two children costs a
total of $${2*(this.costPerNightAdult)+2*(this.costPerNightChild)}.`)
}
}
const safariView = new NationalParkHotels(hotel1)
const lepoardMansion = new NationalParkHotels(hotel2)
safariView.summariseHotel()
lepoardMansion.summariseHotel()
// Another way of doing this is to use a variable for the totalPrice
this.summariseHotel = function(){
const totalPrice = this.costPerNightAdult * 2 + this.costPerNightChild * 2
console.log(`A one night stay at the ${this.name} for two adults and two children costs a total of $${totalPrice}.`)
}
// Object.assign(target, source)
const sandraKayeProfileData = {
name: 'Sandra Kaye',
portfolio: 'www.sandrasportfolio.com',
currentJob: 'Google',
currentSalary: '400k'
}
function DevProfile(data){
Object.assign(this, data)
this.name = data.name
this.portfolio = data.portfolio
this.currentJob = data.currentJob
this.currentSalary = data.currentSalary
this.summariseDev = function(){
console.log(`${this.name}'s porfolio is at ${this.portfolio} and they work at ${this.currentJob}. Their current salary is ${this.currentSalary}.`)
}
}
/*
Challenge
1. Use Object.assign to cut 4 lines of code from the constructor function.
*/
function DevProfile(data){
Object.assign(this, data)
this.summariseDev = function(){
console.log(`${this.name}'s porfolio is at ${this.portfolio} and they work at ${this.currentJob}. Their current salary is ${this.currentSalary}.`)
}
}
const sandraKaye = new DevProfile(sandraKayeProfileData)
sandraKaye.summariseDev()
// Console output:
// Sandra Kaye's porfolio is at www.sandrasportfolio.com and they work at Google. Their current salary is 400k.
Day 124 | 2023-03-17 | Friday | Focus: YarnHelp.app - deploying and redesign |
Main Resources: n/a
Completed:
- See changelog on github repo for dev branch https://github.com/GingerKiwi/yarnhelp/tree/Dev-2023.03
- Woke up at 7am - kept coding until 1pm. It's a flow day!
Refections and Notes:
- Need to refactor to use variables instead of calculations inside string literals for results.
Day 154 | 2023-03-18 | Friday | Focus: YarnHelp.app - Docs |
Main Resources: n/a
Completed: See copy of change log entry from today
Work on Docs and Calculations
-
Figured out that for just the sweater calculations the app will need to do 200 calculations for knit and crochet cardigans in 5 weights of yarn, for 6 sizes including oversized/long, cabled, and cabled cardigans.
- Is it better to pull some of this data (eg total yardage per each project type/size) from a JSON doc?
-
-> Goal is to do the calculations as variables outside of the string literals. The calculations are currently done inside the .innerHTML = statements like below:
babyPulloverAnswer.innerHTML =
`You need ${Number(Math.ceil(550 * 1.05/yardsPerBall.value))} balls of this yarn for a baby pullover`
Instead something like this:
// Worsted Weight (Craft Yarn Council yarn weight 4) for Baby Pullover:
const babyPulloverW4 = Number(Math.ceil(550 * 1.05/yardsPerBall.value))
babyPulloverAnswer.innerHTML = `${babyPulloverW4} balls of this yarn are needed for a baby pullover`
- Add PDF-References Folder to YarnHelp-Docs Folder
- 2 webpages saved as pdf
- Interweave Knits - sweater calculations
- Twisted Yarn Shop - inc crochet, blankets/throws, scarfs, shawls, and accessories
- Craft Yarn Council's Yarn Standards docs
- 2 webpages saved as pdf
- Add Calculations.md
- Start list of yarn requirements for different weights of pullovers and cardigans
- Start list of yarn requirements for blankets and throws
- Add example of calculation in js code block